Using Step Functions to Orchestrate a Series of Lambda Functions
Want to learn more about AWS Lambda and .NET? Check out my A Cloud Guru course on ASP.NET Web API and Lambda.
Download full source code.
This a simple example of using step functions to orchestrate a series of Lambda functions. The Lambda functions execute quickly, return a result, and trigger the next step.
In a follow-up post, I’ll show how to use step functions where a step pauses and needs to be manually resumed at a later time.
This post is not a step-by-step guide, I wrote some earlier posts with more background information, you can look those up.
Overview of the state machine
Though the service is called Step Functions, what you build is referred to as a state machine.
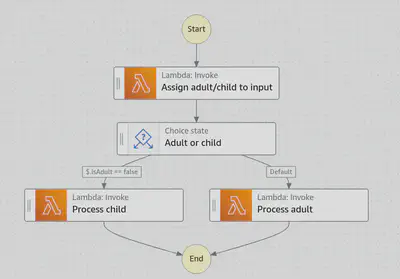
This state machine is a simple one. It uses Lambda functions to decide if the Person
is an adult or a child, then process the person accordingly.
The first step sends the Person
to a Lambda function that determines based on the date of birth whether the person is an adult or a child. The Lambda function returns the Person
with the IsAdult
property set to true
or false
.
The next step examines the IsAdult
property and calls the appropriate Lambda function to process the child/adult, passing the Person
as input.
Definition of the state machine
Here is the definition, you can use this to create the state machine in the AWS console. Name the state machine “ProcessAdultOrChild”. You must replace the account id with your own.
It refers to three Lambda functions, you will see how to create them in the next section.
{
"Comment": "Determine if a person is adult/child and process accordingly",
"StartAt": "Assign adult/child to input",
"States": {
"Assign adult/child to input": {
"Type": "Task",
"Resource": "arn:aws:states:::lambda:invoke",
"OutputPath": "$.Payload",
"Parameters": {
"Payload.$": "$",
"FunctionName": "arn:aws:lambda:us-east-1:012345678910:function:AdultOrChild:$LATEST"
},
"Retry": [
{
"ErrorEquals": [
"Lambda.ServiceException",
"Lambda.AWSLambdaException",
"Lambda.SdkClientException",
"Lambda.TooManyRequestsException"
],
"IntervalSeconds": 2,
"MaxAttempts": 6,
"BackoffRate": 2
}
],
"Next": "Choice"
},
"Choice": {
"Type": "Choice",
"Choices": [
{
"Variable": "$.IsAdult",
"BooleanEquals": false,
"Next": "Process child"
}
],
"Default": "Process adult"
},
"Process child": {
"Type": "Task",
"Resource": "arn:aws:states:::lambda:invoke",
"OutputPath": "$.Payload",
"Parameters": {
"Payload.$": "$",
"FunctionName": "arn:aws:lambda:us-east-1:012345678910:function:ProcessChild:$LATEST"
},
"Retry": [
{
"ErrorEquals": [
"Lambda.ServiceException",
"Lambda.AWSLambdaException",
"Lambda.SdkClientException",
"Lambda.TooManyRequestsException"
],
"IntervalSeconds": 2,
"MaxAttempts": 6,
"BackoffRate": 2
}
],
"End": true
},
"Process adult": {
"Type": "Task",
"Resource": "arn:aws:states:::lambda:invoke",
"OutputPath": "$.Payload",
"Parameters": {
"Payload.$": "$",
"FunctionName": "arn:aws:lambda:us-east-1:012345678910:function:ProcessAdult:$LATEST"
},
"Retry": [
{
"ErrorEquals": [
"Lambda.ServiceException",
"Lambda.AWSLambdaException",
"Lambda.SdkClientException",
"Lambda.TooManyRequestsException"
],
"IntervalSeconds": 2,
"MaxAttempts": 6,
"BackoffRate": 2
}
],
"End": true
}
}
}
The Lambda functions
The Lambda functions are simple (see the attached zip).
The first AdultOrChild
takes in a Person
and returns a Person
with the Age
and IsAdult
properties set.
The other two, ProcessChild
and ProcessAdult
take in the Person
. They return a Response
with a message and a bool indicating if the person is an adult or child.
Deploy the three functions with the names mentioned here.
For instructions on deploying Lambda functions, see this post
Testing the state machine
You can test the state machine in the AWS console. Open the ProcessAdultOrChild
state machine then click Start Execution.
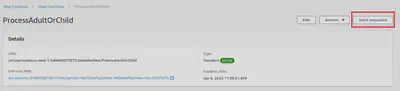
Paste in JSON and click Start Execution. The JSON should look like this:
{
"FirstName": "Melissa",
"LastName": "Conner",
"DateOfBirth": "2000-11-09"
}
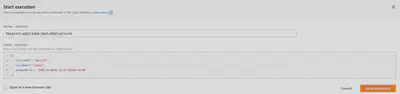
This will kick off the state machine.
Then you will see the execution complete.
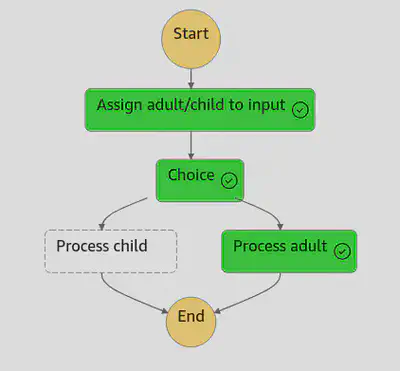
Try with a different date of birth to see the state machine execute the other path.
Conclusion
Step functions and state machines are a powerful way to orchestrate a series of Lambda functions. This example showed how to orchestrate a series of functions that respond quickly.
In a follow-up post, I will show how a state machine can be paused while a long-running task is performed. Then the state machine can be resumed when the long-running task is complete.
Download full source code.