Simple Lambda Function with a Function URL
Want to learn more about AWS Lambda and .NET? Check out my A Cloud Guru course on ASP.NET Web API and Lambda.
Download full source code.
This blog post shows a simple way to create a Lambda function that uses a Function URL. I will reference this blog post in more complicated posts later.
Getting the tools
Install the latest tooling, this lets you deploy and run Lambda functions.
dotnet tool install -g Amazon.Lambda.Tools
Install the latest templates.
dotnet new --install Amazon.Lambda.Templates
Get the latest version of the AWS CLI, from here.
1. Create the Lambda function
From the command line, create a new Lambda function using the lambda.EmptyFunction
template -
dotnet new lambda.EmptyFunction --name SimpleLambdaWithFunctionUrl
2. Edit the code to handle HTTP requests
The lambda.EmptyFunction
template does not support HTTP requests, but it is easy to fix this.
Change to the SimpleLambdaWithFunctionUrl/src/SimpleLambdaWithFunctionUrl
directory.
Add the package Amazon.Lambda.APIGatewayEvents
-
dotnet add package Amazon.Lambda.APIGatewayEvents
Add the following using statements -
using System.Net;
using System.Text.Json;
using Amazon.Lambda.APIGatewayEvents;
Change the FunctionHandler(..)
method to this -
public APIGatewayHttpApiV2ProxyResponse FunctionHandler(APIGatewayHttpApiV2ProxyRequest request, ILambdaContext context)
{
var response = new APIGatewayHttpApiV2ProxyResponse
{
StatusCode = (int)HttpStatusCode.OK,
Body = $"{JsonSerializer.Serialize(request)}" , // serialize the request and return it
Headers = new Dictionary<string, string> { { "Content-Type", "application/json" } }
};
return response;
}
The above code takes in an APIGatewayHttpApiV2ProxyRequest
and returns an APIGatewayHttpApiV2ProxyResponse
.
The response body includes a serialized version of the request.
3. Deploy the Lambda function
Use the following to build the code and deploy the Lambda function -
dotnet lambda deploy-function SimpleLambdaWithFunctionUrl
You will then be asked - “Enter name of the new IAM Role:”, put in “SimpleLambdaWithFunctionUrlRole”.
Then you will be asked to - “Select IAM Policy to attach to the new role and grant permissions”, select “AWSLambdaBasicExecutionRole”, for me it is number 6 on the list.
Wait as the function and permissions are created.
4. Configuring the function for HTTP requests
Right now, the Lambda function is deployed and can be seen in the AWS Console, but it doesn’t have a Function URL.
You can add one from the AWS Console or the AWS CLI (or other tools). But I want to use the AWS CLI, a minimum version of “2.5.4” is required (see above on tooling).
From the command line, run -
aws lambda create-function-url-config --function-name SimpleLambdaWithFunctionUrl --auth-type NONE
You will see -
{
"FunctionUrl": "https://xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx.lambda-url.us-east-1.on.aws/",
"FunctionArn": "arn:aws:lambda:us-east-1:xxxxxxxxxxx:function:SimpleLambdaWithFunctionUrl",
"AuthType": "NONE",
"CreationTime": "2022-08-25T16:21:11.719531Z"
}
At this point, a URL is attached to the Lambda function, requiring no authentication, but you’re not quite finished yet.
5. Add a resource-based policy to allow the Function URL to invoke the Lambda function
One more thing to do - add a resource-based policy allowing the Lambda function to be called via the URL. See here for more.
aws lambda add-permission --function-name SimpleLambdaWithFunctionUrl --statement-id AuthNone --action lambda:InvokeFunctionUrl --principal * --function-url-auth-type NONE
You will get a response like -
{
"Statement": "{"Sid":"AuthNone","Effect":"Allow","Principal":"*","Action":"lambda:InvokeFunctionUrl","Resource":"arn:aws:lambda:us-east-1:xxxxxxxxx:function:SimpleLambdaWithFunctionUrl","Condition":{"StringEquals":{"lambda:FunctionUrlAuthType":"NONE"}}}"
}
Now the Lambda function can be accessed from the URL with no authentication needed.
If you are interested in using IAM Auth when making a request to a Lambda function, see this post.
6. Call the Lambda function
Open the URL you got in step 4, in a web browser.
The request you sent is serialized and returned to you.
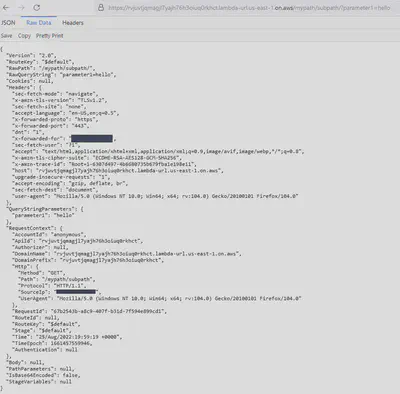
Download full source code.