Lambda Function URLs with .NET 6 and CORS
Want to learn more about AWS Lambda and .NET? Check out my A Cloud Guru course on ASP.NET Web API and Lambda.
Download full source code.
I have recently written posts on .NET and Lambda Function URLs, and a simple CORS example with .NET 6.
In this post, I’m going to combine the two ideas and show you how to make requests from a .NET 6 web application to a .NET 6 Lambda function that is accessible via a Lambda Function URL.
Keep in mind, CORS is a large and complex topic. Your needs may be well beyond the simple solution shown here.
tl;dr just show me the commands!
1. Create the Lambda Function with Function URL
Follow all the steps in my Lambda Function URLs post) to create the Lambda function and to make it accessible via a Function URL.
BUT replace the FunctionHandler(..)
code with the following -
public Person FunctionHandler(APIGatewayProxyRequest request, ILambdaContext context)
{
Person person = new Person { FirstName = "Jane", LastName = "Doe", Age = 42 };
return person;
}
Deploy this function and you should be able to access it via the function URL in your browser, CURL, Postman, etc. You will get -
{"FirstName":"Jane","LastName":"Doe","Age":42}
2. Create the .NET 6 web application
Create a web application (dotnet new webapp --name ...
).
Open Pages/index.cshtml
and replace with the following code -
@page
<div class="text-center">
<h1>A very simple CORS demo</h1>
</div>
<input type="button" value="Call Lambda Function URL" onclick="FetchRemote('https://xxxxxxxxxxxx.lambda-url.us-east-1.on.aws','/', 'GET')"/>
<div>
<span id='remoteResponse'></span>
</div>
<script src="~/js/site.js"></script>
Replace the https://xxxxxxxxxxxx.lambda-url.us-east-1.on.aws
with the URL of the Lambda function you created in step 1.
As you can tell frontend dev is not my thing, same goes for the JavaScript below.
Open the wwwroot/js/site.js
file and add the following code -
function FetchRemote(remoteHost, path, httpMethod) {
const remoteResponse = document.getElementById('remoteResponse');
fetch(`${remoteHost}${path}`,
{
method: httpMethod,
}).then(response => {
if (response.ok) {
response.text().then(text => {
remoteResponse.innerText = text;
});
}
else {
remoteResponse.innerText = response.status;
}
})
.catch(() => remoteResponse.innerText = 'An error occurred, might be CORS?! :) Press F12 to open the web debug tools');
}
Open Properties/launchSettings.json
, there will be a profile with the name of the application you created, change the ApplicationUrl
to https://localhost:8081/
.
Your web application should now be able to call your Lambda function URL using JavaScript.
3. Try to call the Lambda function URL from the web application
Start the web application and try to call the Lambda function URL by clicking the “Call Lambda Function URL” button.
You will get a CORS error.
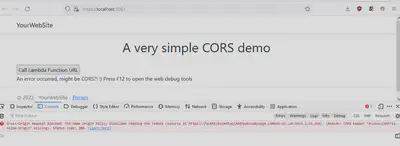
This is expected.
4. Add CORS to the Lambda Function URL
The fix has to be made in the Lambda function. The function needs to allow CORS requests from the web application.
With the AWS CLI, it is very easy to make the change to the Lambda function.
Run the following command -
aws lambda update-function-url-config --function-name FunctionUrlExample --cors AllowOrigins=https://localhost:8081
You will see output like this -
{
"FunctionUrl": "https://xxxxxxxxxxxxxx.lambda-url.us-east-1.on.aws/",
"FunctionArn": "arn:aws:lambda:us-east-1:xxxxxxxxxx:function:FunctionUrlExample",
"AuthType": "NONE",
"Cors": {
"AllowOrigins": [
"https://localhost:8081"
]
},
"CreationTime": "2022-04-14T18:30:24.400146Z",
"LastModifiedTime": "2022-04-28T20:04:26.879033Z"
}
5. Retry calling the Function URL from the web application
Wait a few seconds for the CORS changes to take effect.
Then click the “Call Lambda Function URL” button in the browser again.
This time it should succeed.
If it doesn’t, make sure that your web application is running on the port you specified in the command in step 4.
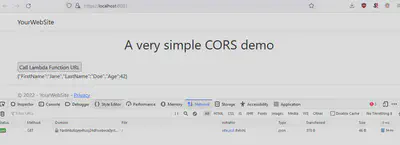
That’s it! You can now call the Lambda function URL from the web application.
Download full source code.