.NET 6 Lambdas on ARM64 - Part 1, Functions
Want to learn more about AWS Lambda and .NET? Check out my A Cloud Guru course on ASP.NET Web API and Lambda.
Download full source code.
AWS Lambda allows you to run your code using x86_64 and ARM64 processors. The default Lambda templates use x86_64, but it is very easy to change these to use ARM64.
I have a few other posts on running .NET 6 on AWS Lambda.
Getting the tools
Install the latest tooling, this lets you deploy and run Lambdas.
dotnet tool install -g Amazon.Lambda.Tools
Install the latest templates to get .NET 6 support.
dotnet new --install Amazon.Lambda.Templates
A little background
To see a list of the available Lambda templates run -
dotnet new lambda --list
You will see a list like this -
Template Name Short Name Language Tags
---------------------------------------- -------------------------------------------- -------- ---------------------
Empty Top-level Function lambda.EmptyTopLevelFunction [C#] AWS/Lambda/Serverless
Lambda ASP.NET Core Minimal API serverless.AspNetCoreMinimalAPI [C#] AWS/Lambda/Serverless
Lambda ASP.NET Core Web Application w... serverless.AspNetCoreWebApp [C#] AWS/Lambda/Serverless
Lambda Custom Runtime Function (.NET 6) lambda.CustomRuntimeFunction [C#],F# AWS/Lambda/Function
Lambda Detect Image Labels lambda.DetectImageLabels [C#],F# AWS/Lambda/Function
Lambda Empty Function lambda.EmptyFunction [C#],F# AWS/Lambda/Function
Lambda Empty Function (.NET 6 Contain... lambda.image.EmptyFunction [C#],F# AWS/Lambda/Function
Lambda Empty Serverless serverless.EmptyServerless [C#],F# AWS/Lambda/Serverless
Lambda Empty Serverless (.NET 6 Conta... serverless.image.EmptyServerless [C#],F# AWS/Lambda/Serverless
Lambda Giraffe Web App serverless.Giraffe F# AWS/Lambda/Serverless
Lambda Simple Application Load Balanc... lambda.SimpleApplicationLoadBalancerFunction [C#] AWS/Lambda/Function
Lambda Simple DynamoDB Function lambda.DynamoDB [C#],F# AWS/Lambda/Function
Lambda Simple Kinesis Firehose Function lambda.KinesisFirehose [C#] AWS/Lambda/Function
Lambda Simple Kinesis Function lambda.Kinesis [C#],F# AWS/Lambda/Function
Lambda Simple S3 Function lambda.S3 [C#],F# AWS/Lambda/Function
Lambda Simple SNS Function lambda.SNS [C#] AWS/Lambda/Function
Lambda Simple SQS Function lambda.SQS [C#] AWS/Lambda/Function
Lex Book Trip Sample lambda.LexBookTripSample [C#] AWS/Lambda/Function
Order Flowers Chatbot Tutorial lambda.OrderFlowersChatbot [C#] AWS/Lambda/Function
Note how some of the templates start with lambda
and some with serverless
. A single configuration change is needed to deploy to ARM64, but the change depends on the template you are using.
lambda vs serverless templates
Applications built from lambda.*
templates are invoked by sending JSON requests to them, directly, from other applications, or from other services.
Applications built from serverless.*
templates are invoked by sending HTTP requests to them via an API Gateway.
Moving to ARM64
If you are using the lambda.
templates, a change is needed to aws-lambda-tools-defaults.json
, covered in this post.
If you are using the serverless.
templates, a change is needed to the serverless.templates
file and will be covered in the next post.
Create the application with the lambda.* template
Create a simple Lambda function with the lambda.EmptyFunction
template -
dotnet new lambda.EmptyFunction --name LambdaEmptyFunctionArm64
Edit the deployment configuration
Navigate to the LambdaEmptyFunctionArm64\src\LambdaEmptyFunctionArm64
directory.
Open the aws-lambda-tools-defaults.json
file and add this -
"function-architecture": "arm64",
Your file should look something like -
{
"Information": [
"snip.."
],
"profile": "",
"region": "",
"configuration": "Release",
"function-runtime": "dotnet6",
"function-architecture": "arm64",
"function-memory-size": 256,
"function-timeout": 30,
"function-handler": "LambdaEmptyFunctionArm64::LambdaEmptyFunctionArm64.Function::FunctionHandler"
}
Update the FunctionHandler method
Open Function.cs
and replace the FunctionHandler(..)
method with this -
public string FunctionHandler(string input, ILambdaContext context)
{
var architecture = System.Runtime.InteropServices.RuntimeInformation.ProcessArchitecture;
var dotnetVersion = Environment.Version.ToString();
return $"Architecture: {architecture}, .NET Version: {dotnetVersion} -- {input?.ToUpper()}";
}
Build
Try building the code before moving on to deploying the application.
Deploy
Deploy using -
dotnet lambda deploy-function --functionname LambdaEmptyFunctionArm64
You’ll be asked which IAM role you want to use, the last number on the list will be for *** Create new IAM Role ***
, pick that.
Then enter a name for the role, something like LambdaNet6_role
will do.
Finally, you’ll be asked what permissions to grant, select AWSLambdaRole
(it is number 9 on my list).
It will then take a moment or two to create the role.
That’s it, you now have a .NET 6 Lambda function running on ARM64 processors.
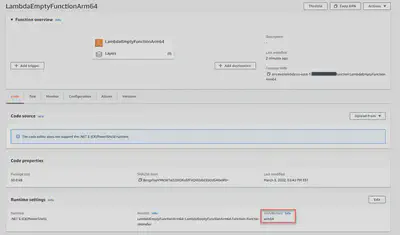
Running the Lambda
You can test the function with the following -
dotnet lambda invoke-function LambdaEmptyFunctionArm64 --payload "hello arm64"
You should get a response that includes this -
Payload:
"Architecture: Arm64, .NET Version: 6.0.1 -- HELLO ARM64"
Download full source code.