A Few (too many?) Ways of Setting the Kestrel Ports in .NET 6
Full source code available here
I was trying to figure out how to programmatically determine what ports Kestrel is running on, but along the way, I found many different ways to set the ports for Kestrel, and I’m sure there are more.
My project was created using -
dotnet new webapi --name AFewWaysToSetKestrelPorts
Here are some of the ways to set the Kestrel ports.
launchSettings.json
The launchSettings.json
file is used when you start the application from Visual Studio or VS Code. Within this file there are two profiles.
The AFewWaysToSetKestrelPorts
profile will launch the application on ports 3000, and 3001. In VS Code all you need to do is press F5. In Visual Studio you need to select “AFewWaysToSetKestrelPorts” from the list, then press F5.
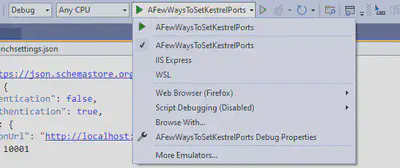
The second profile IIS Express
relates to the ports IIS Express will use from inside Visual Studio when that option is selected. In my example, it will use ports 10000 and 10001.
appsettings.json
You can set the Kestrel ports inside the appsettings.json
file.
A simple block of JSON does it.
{
"Kestrel": {
"Endpoints": {
"Https": {
"Url": "https://*:8001"
},
"Http": {
"Url": "http://*:8000"
}
}
}
}
You might notice that there is an appsettings.Development.json
, I’m going to write another post about this in a few days.
IWebHostBuilder.UseKestrel
At the top of Program.cs
file you can add the following -
var builder = WebApplication.CreateBuilder(args);
builder.WebHost.UseKestrel(serverOptions =>
{
serverOptions.ListenAnyIP(4000);
serverOptions.ListenAnyIP(4001, listenOptions => listenOptions.UseHttps());
});
IWebHostBuilder.UseUrls
A little ways down the Program.cs
file, after the call to builder.Build()
you can add use .UseUrls(..)
.
//snip...
builder.Services.AddEndpointsApiExplorer();
builder.Services.AddSwaggerGen();
var app = builder.Build();
builder.WebHost.UseUrls("http://*:6000;https://*:6001");
//snip...
Note, these URLs will be overridden by the settings in the appsettings.json
file.
WebApplication.Urls.Add
Like the above one, after the call to `builder.Build()` you can use `.Urls.Add(..)`.
//snip...
builder.Services.AddEndpointsApiExplorer();
builder.Services.AddSwaggerGen();
var app = builder.Build();
app.Urls.Add("http://*:7000");
app.Urls.Add("https://*:7001");
//snip...
Note, these URLs will also be overridden by the settings in the appsettings.json
file.
Command line
dotnet run --urls "http://*:11000;https://*:11001"
This will be overridden by settings in appsettings.json
file.
Environment variable
Per the suggestion from Dalibor, here is a way to set the port from environment variables.
Set ASPNETCORE_URLS
to something like http://localhost:15000;https://localhost:15001
.
Depending on you OS and Shell there are a variety of ways, here is how do it in PowerShell -
$Env:ASPNETCORE_URLS = "http://localhost:15000;https://localhost:15001"
And command prompt -
set ASPNETCORE_URLS=http://localhost:15000;https://localhost:15001
Overriding
There are more nuanced overriding rules than laid out here, you should experiment to make sure it works the way you expect.
Full source code available here